URL/URI Encode and Decode Tool
Encodes or decodes a string to support Uniform Resource Identifier (URI)
Easily escape and unescape strings using encodeURIComponent, encodeURI, decodeURIComponent, decodeURI functions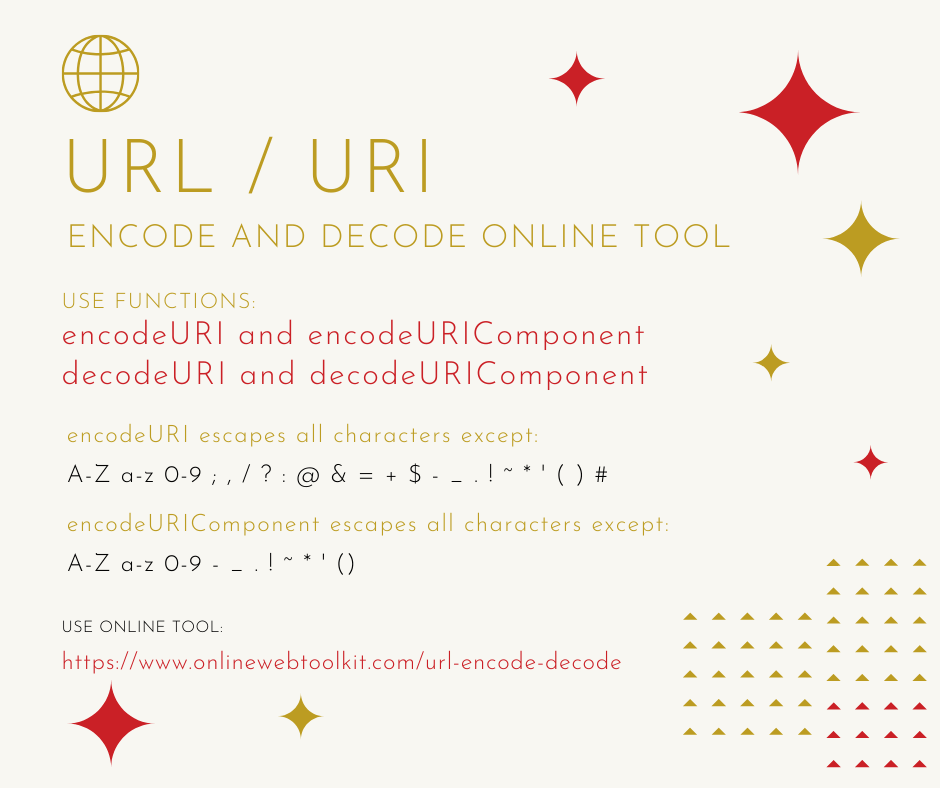
Online URL Encode and Decode Tool
URL encoding converts characters into a format that can be transmitted over the Internet. URLs can only be sent over the Internet using the ASCII character-set. Since URLs often contain characters outside the ASCII set, the URL has to be converted into a valid ASCII format. URL encoding replaces unsafe ASCII characters with a "%" followed by two hexadecimal digits. URLs cannot contain spaces. URL encoding normally replaces a space with a plus (+) sign or with %20.
What is URL Encoding and URL Decoding?
URL encoding is a process of converting special characters in a URL into a format that can be transmitted over the internet. It replaces characters that have a special meaning in a URL with a sequence of characters that is safe to transmit. URL decoding is the reverse process of URL encoding, where a sequence of characters that were previously encoded using URL encoding are converted back to their original form.
For example, suppose you want to send a URL that contains a space character. A space character is not valid in a URL and will cause an error if not encoded. In this case, URL encoding replaces the space character with "%20". When the URL is received, it is decoded to its original form, which is a space character.
URL encoding and decoding are often used in web applications to handle special characters in a URL, such as spaces, commas, and other symbols.
Here are some examples of URL encoding and decoding:
URL encoding:
Original text: Hello, world! Original text: http://onlinewebtoolkit.com/?query=hello world
Encoded text: Hello%2C%20world%21
Encoded text: http%3A%2F%2Fonlinewebtoolkit.com%2F%3Fquery%3Dhello%20world
URL decoding:
Encoded text: Hello%2C%20world%21 Encoded text: http%3A%2F%2Fonlinewebtoolkit.com%2F%3Fquery%3Dhello%20world
Decoded text: Hello, world!
Decoded text: http://onlinewebtoolkit.com/?query=hello world
Similarly, other special characters like "&", "/", ":", ";", "=", "?", "@", "#", "<", ">", "{", "}", "|", "^", "~", "[", "]", and "`" are replaced with their respective percent-encoded values.
URL Encoding and Decoding are commonly used in web programming, where URLs are frequently used to pass data between web pages, applications, and servers.
Reasons to URL Encoding and Decoding
Here are some examples of when URL encoding and decoding can be used:
URL encoding:
- To encode special characters in a URL such as spaces, quotes, and question marks.
- To include non-ASCII characters in a URL, such as accented letters or characters from other languages.
- To pass data between web pages via query parameters in a URL.
- Encoding data in APIs or web services.
- Encoding data in form submissions, especially when using GET method.
URL decoding:
- To decode query parameters passed in a URL and extract the data.
- To decode data that has been encoded in a URL-safe format, such as Base64-encoded data.
- To decode URLs that have been mistakenly encoded multiple times, resulting in a URL that doesn't work.
- Decoding URL-encoded data in web scraping or data extraction.
- Decoding URL-encoded data received from a form or API.
- Decoding URL-encoded data in JavaScript or other programming languages.
- Decoding URLs to display them in a human-readable format.
encodeURI
encodeURI is a function in JavaScript that is used to encode a complete URI/URL by replacing all non-ASCII characters and certain ASCII characters with a percent (%) sign followed by a two-digit hexadecimal code.
encodeURI escapes all characters except: A-Z a-z 0-9 ; , / ? : @ & = + $ - _ . ! ~ * ' ( ) #
The syntax for encodeURI is as follows:
encodeURI(uri)
Here, uri is the complete URI that needs to be encoded.
For example, the URI "https://www.onlinewebtoolkit.com/page with spaces.html" can be encoded using `encodeURI` as follows:
encodeURI("https://www.onlinewebtoolkit.com/page with spaces.html")
This would result in the following encoded URI:
"https://www.onlinewebtoolkit.com/page%20with%20spaces.html"
Note that the spaces in the original URI have been replaced with `%20`, which is the hexadecimal escape sequence for a space character.
`encodeURI` should be used to encode a URI or an existing URL.
encodeURIComponent
encodeURIComponent is a function in JavaScript that encodes a Uniform Resource Identifier (URI) component by replacing each instance of certain characters with one, two, three, or four escape sequences representing the UTF-8 encoding of the character. The characters that are not encoded are ASCII alphanumeric characters and certain reserved characters.
encodeURIComponent escapes all characters except: A-Z a-z 0-9 - _ . ! ~ * ' ()
The syntax of the encodeURIComponent function is:
encodeURIComponent(uriComponent)
where uriComponent is a string that represents the URI component to be encoded.
The `encodeURIComponent` function is typically used to encode the values of query string parameters in a URL. This is necessary because the values may contain characters that have special meaning in a URL, such as `&`, `?`, and `=`. By encoding these characters, they can be safely included in a URL without affecting its structure.
For example, the following code uses `encodeURIComponent` to encode the value of the q parameter in a Google search URL:
let searchTerm = "JavaScript tutorial";
let url = "https://www.google.com/search?q=" + encodeURIComponent(searchTerm);
console.log(url);
The output of this code will be:
https://www.google.com/search?q=JavaScript%20tutorial
where `%20` represents the encoded space character.
`encodeURIComponent` should be used to encode a URI Component - a string that is supposed to be part of a URL.
decodeURI
`decodeURI()` is a built-in function in JavaScript that is used to decode a URI (Uniform Resource Identifier) that has been previously encoded with the `encodeURI()` function. It converts the encoded characters in the URI back to their original characters.
Here is an example of using decodeURI():
const encodedUri = "https://en.wikipedia.org/wiki/Caf%C3%A9";
const decodedUri = decodeURI(encodedUri);
console.log(decodedUri); // Output: "https://en.wikipedia.org/wiki/Café"
In this example, the encoded URI "https://en.wikipedia.org/wiki/Caf%C3%A9" is decoded using `decodeURI()`, which converts the "%C3%A9" back to "é".
decodeURIComponent
`decodeURIComponent()` is a built-in JavaScript function used to decode a Uniform Resource Identifier (URI) component previously created by the `encodeURIComponent()` function.
It takes a single parameter, which is the URI component to decode, and returns the decoded string.
Here is an example:
const encodedURI = 'https://example.com/%2Fpage%2F1%3Fname%3DJohn%26age%3D30';
const decodedURI = decodeURIComponent(encodedURI);
console.log(decodedURI);
// Output: https://example.com//page/1?name=John&age=30
In this example, the `encodedURI` variable contains a URI component that has been encoded using `encodeURIComponent()`. The `decodeURIComponent()` function is then used to decode the URI component, resulting in the `decodedURI` variable containing the original, decoded string.
The decodeURI and decodeURIComponent function decodes a Uniform Resource Identifier (URI) component previously created by encodeURIComponent or by a similar routine. Replaces each escape sequence in the encoded URI component with the character that it represents.
How to Use the URL/URI Encode and Decode Tool?
- Browse or open URL/URI Encode and Decode Tool - https://www.onlinewebtoolkit.com/url-encode-decode
- Paste your URL or string in the "Plain Text" field.
- Press the “Encode URI Component” or "Encode URI" button.
- You will get your output in a moment in the URL Encoded output textbox.
- Download or copy the result from the "URL Encoded" field.
- Paste your encoded string in the "Encoded" text field.
- Press the “Decode URI Component” and "Decode URI" button.
- You will get your output in a moment in the plain text output textbox.
- Download or copy the result from the 1st or decoded "Text" field.
- You can use options as "Copy to Clipboard", "Download", and "Clear" options.
- Alternatively, you can download encoded/decoded text by simply clicking on the "Download" button.
How to convert URL/URI Encode online
How to convert URL/URI Decode online
Useful Features of Our Online URL/URI Encode and Decode Tool
Free and Simple to Use
The use of this tool comes at no cost, and it's effortless to use. With the simple set of instructions provided, you'll be able to view and run codes easily.
Compatibility
This tool is a cloud-based utility and supported by all operating systems, including iOS, Android, Windows, and Mac OS, allowing you to access and use it for viewing HTML files from any device.
No Plugin Installation Needed
You can access this tool through the web browser of your device without having to install any plugins. This HTML viewer operates without the need for any plugins, making it convenient to use.
Speedy and Secure
The tool displays results on the user's screen in mere seconds, and it's a secure online utility that doesn't save any data entered or uploaded by users in its databases.
Accessible from Everywhere
You can access our tool from anywhere in the world as long as you have an internet connection. Simply connect your device to the internet, and you'll be able to use and access this code viewer.
Privacy of Users’ Data
At Onlinewebtoolkit, we offer a variety of online tools, including an URL/URI Encode and Decode Tool, and we take the privacy of our users' data very seriously. With so many online scams, many people are concerned about their sensitive information being compromised when using online tools. However, our website provides a secure and safe tool that prevents hackers from accessing or intentionally sharing users' information with third parties. The text you input into our tool is only stored temporarily on the client side within your browser until the formatting process is complete. Once the results are displayed or you refresh or close the browser, your data is deleted from our site.